Books in this series are published only by invitation of a member of the editorial board. Abstracts are written based on an invitation from a member of the editorial board.
The First Example: Hello, World!
While it works well as a beginner language, Python is also suitable for more advanced tasks and is currently one of the most widely used programming languages in the world. For example, in the popular Spyder IDE, the editor is usually located in the upper left window, while the lower right corner window is the iPython window where you run the program.2.
Different Ways to Use Python
In Python 2, the program above would print "Hello, World!" read, that is, without the brackets. Since almost all code examples use some type of printing, programs written in Python 2 will usually not run in Python 3.
Programming Simple Mathematics
Because using parentheses to group calculations works exactly the same as in math, it's not that hard to understand for those with a math background. However, when programming more complicated formulas, it is very easy to make mistakes, such as forgetting or misplacing a closing parenthesis.
Variables and Variable Types
In these statements, the expression on the right side of the equals sign is evaluated first, and then the result is assigned to the variable on the left. The right side is first evaluated using the value of already defined and then the t variable is updated to hold the result of the calculation.
Formatting Text Output
When Python encounters curly braces within an f-string, it will evaluate the contents of the curly braces, which can be an expression or a variable, and insert the resulting value into the string. For example, we may want to control the number of decimal places when outputting numbers.
Importing Modules
Why not use the simple import from math * and gain access to all the math functions we need. Commandpydoc in a terminal window can be used to list information about a module (try, for example, pydoc math), or we can import the module into a Python program and list its contents with the builtin function.
Pitfalls When Programming Mathematics
In Python 2 and many other programming languages, accidental division of integers can sometimes produce surprising results. In this chapter, we will introduce the concept of loops, which can be used to automate repetitive and tedious operations.
Loops for Automating Repetitive Tasks
In addition to the loop concept, we will introduce Boolean expressions, which are true/false expressions, and a new type of variable called list, which is used to store sequences of data. In a loop like this, all the lines we want to repeat within the loop must be indented, with the same indentation.
Boolean Expressions
Most of the Boolean expressions we'll use in this course are of the simple kind above, consisting of a single equation that should be known from mathematics. The rules for evaluating such compound expressions are as expected: C1 and C2 is true if both C1 and C2 are true, while C1 or C2 is true if at least one of the two conditions C1 and C2 is true.
Using Lists to Store Sequences of Data
The last line uses the built-in Python function that returns the number of elements in the list. If we actually want to make a copy of the original list, we must specify this explicitly with b = a.copy().
Iterating Over a List with a for Loop
The for loop will simply iterate over a given list, perform the operations we want on each element, and then stop when it reaches the end of the list. Better alternatives include a built-in Python function called range, often in combination with a for loop or a so-called list comprehension.
Nested Lists and List Slicing
As for the array function, we can omit some of the arguments and rely on default values. As for the nested lists considered above, a good way to get familiar with list slicing is to create a small.
Tuples
In diesem Kapitel enthaltene Bilder und anderes Material Dritter unterliegen ebenfalls der genannten Creative Commons-Lizenz, sofern in der Bildlegende nichts anderes angegeben ist. Sofern das betreffende Material nicht unter die genannte Creative-Commons-Lizenz fällt und die betreffende Handlung nach den gesetzlichen Bestimmungen nicht zulässig ist, ist für die weitere Nutzung des oben aufgeführten Materials die Zustimmung des jeweiligen Rechteinhabers einzuholen.
Programming with Functions
Starting with the first line, def amount(n): is called the function header, and defines the function's interface. Code inside function definitions is not executed until we include a call to the function in the main program.
Function Arguments and Local Variables
Similarly, if we want the function to change a global variable, then we have to make the function return this variable, instead of using the global keyword. Note that, here, we return two values from the function, separated by commas, just like in the argument list, and we also assign the return values to the global variable0, on the line where the function is called.
Default Arguments and Doc Strings
Doc strings don't take much time to write and are very useful for others who want to use the function. Much of the online Python library and module documentation is automatically generated from doc strings included in the code.
If-Tests for Branching the Program Flow
The conditions are checked one by one, and as soon as one evaluates to true, the corresponding block is executed and the program goes to the first statement after the second block. If neither condition is true, the code inside the else block is executed.
Functions as Arguments to Functions
One answer is that the lambda function definition can be placed directly in the argument list of the other function. Using lambda functions in this way can be very convenient in cases where we need to pass a simple mathematical expression as an argument to a Python function.
Solving Equations with Python Functions
Like the halving method, Newton's method is easy to implement in a while loop, and we can implement it as a generic function that takes a Python function implementingf(x) as an argument. Newton's method usually converges much faster than the halving method, but has the disadvantage that the functional must be differentiated manually.
Writing Test Functions to Verify our Programs
For the smaller programs we write in this course, it can be just as easy to write the test functions in the same file as the functions being tested. This statement should be removed once we know the feature is working correctly and as we get used to how the test features work.
Reading User Input Data
If we want a different value of a variable, we have to modify the code and rerun the program. The first element, sys.argv[0] is the name of the .py file that contains the program.
Flexible User Input with eval and exec
While the program is running, the user is first asked to write a formula, which turns into a function. Then the user is prompted for x values until the answer is None, and the program evaluates the function f(x) for eachx.
Reading Data from Files
For each pass through the for loop, a single line of the file is read and stored in the string variable, and inside the for loop we add any code we want to process that line. Returning to the concrete data file above, let's say the only processing we want is to calculate the mean of the numbers in the file.
Writing Data to Files
The innermost loop loops through each row, writing the numbers to the file one at a time. After this line, a pass through the outer for loop ends and the program moves on to the next iteration and the next row in the table.
Handling Errors in Programs
The structure of the nested for loop is also worth reviewing in the above code. If there are no errors in the try block, the code in the except block is not executed and the program continues to the first line after the try except block.
Making Modules
The solution is to add such sample code in a test block at the end of the module file. On Unix-like systems (Linux, Mac, etc.), the standard way to tell Python where to look is by modifying the environment variable called PYTHONPATH.
NumPy and Array Computing
You've probably used a number of different drawing tools in the past, and we're now going to do much of the same in Python. Now that we have the two lists, they can be sent directly to a tool like matplotlib for plotting, but before we do that, we'll introduce NumPy arrays.
Plotting Curves with Matplotlib
The last two lines perform the actual plotting: the plt.plot(x,y) call first creates the curve plot, and then plt.show() displays the plot on the screen. Most of the lines in the code should be self-explanatory, but a few are worth commenting out.
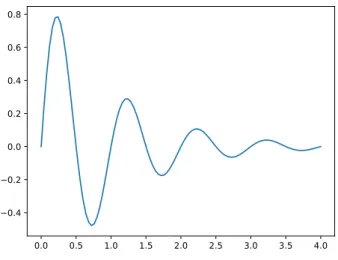
Plotting Discontinuous and Piecewise-Defined Functions
We see that this last approach ensures that we can call the function with an array argumentx, but the downside of both versions is that we have to write a lot of new code, and using a for loop is much slower than using vectorized set calculus. For this particular case, the NumPy function where will evaluate the expression nx<0 for all elements in the array, and return an array of the same length asx, with values 0.0 for all elements kux<0 and 1.0 for the others.
Making a Movie of a Plot
This approach is the simplest of the three and requires very few tools that we haven't seen before. We simply use a for loop to loop through the values, calculating the new y-values and updating the graph for each iteration of the loop.

More Useful Array Operations
We can create an array of any dimension by passing in a tuple of the appropriate length. It is therefore often useful to use zeros to create an array of the correct size and then fill in the non-zero values.
Dictionaries
Dictionaries can be initialized in two different ways: One is by using the curly brackets, as in the example above. There may be applications where it is important to sort the keys in this way, but usually the order of a dictionary is unimportant.
Example: A Dictionary for Polynomials
Since the keys in the polynomial dictionary are integers, we can also replace the dictionary with a list, where the list index corresponds to the power of the respective term. The function to evaluate a polynomial represented by a list is almost identical to the function for the dictionary.
Example: Reading File Data to a Dictionary
String Manipulation
In the last example, we combine the country finder to replace all the text before the ":" with "Bonn". In most of the examples reviewed so far, we've mostly used splits to process line-by-line text files, but in some cases we have a string of a.
Basics of Classes
The constructor creates and returns an instance of the class with the specified values of the parameters, and we assign this instance to the variable b. In this version of the class, the other variables are regular local variables defined within the method.
Protected Class Attributes
While it may be tempting to adjust a bank account balance when needed, this is not the intended use of the class. This convention tells other programmers that a given property or method is not supposed to be accessed from outside the class, even though it is still technically possible to do so.
Special Methods
The actual content of the special method is in all cases entirely up to the programmer. We see that the list contains the same default (mostly useless) versions of the special methods, but some of the items are more meaningful.
Example: Automatic Differentiation of Functions
For many functions f(x), finding f(x) can require time-consuming and tedious derivations, and in such cases the derivation class comes in very handy:.
Test Functions for Classes
The function is defined to take one argument xd also using two local variables and b that are defined outside the function before it is called. The answer is that a function defined inside another function "remembers" or has access to all local variables of the function where it is defined.
Example: A Polynomial Class
The order of the sum of the two polynomials is equal to the highest order of both, so the length of the returned polynomial must be equal to the length of the longest of the dycoeff lists. We use this knowledge in the code by starting with a copy of the longest list and then looping through the shorter ones and adding to each element.
Class Hierarchies and Inheritance
To make the class represent a parabola, we need to add the missing code, that is, the code that changes between Line and Parabola. When we use the class name directly, we have to include ourselves as the first argument, while this aspect is handled automatically when we use super().
Example: Classes for Numerical Differentiation
In this case, we can create a superclass that contains the constructor and let the different subclasses implement their own version of the __call__ method. We can empirically examine the accuracy of the numerical differentiation formulas using the class hierarchy created above.
Example: Classes for Numerical Integration
Alternatively, we can of course not include the construct_method method in the superclass at all. The superclass provides a common framework for implementing the various methods, which can then be realized as subclasses.