Although this book maintains the same pedagogical approach and general structure of Data Structures and Algorithms in Java, the code fragments have been completely redesigned. Furthermore, this course sequence is typically followed at a later point in the curriculum by a more in-depth study of data structures and algorithms.
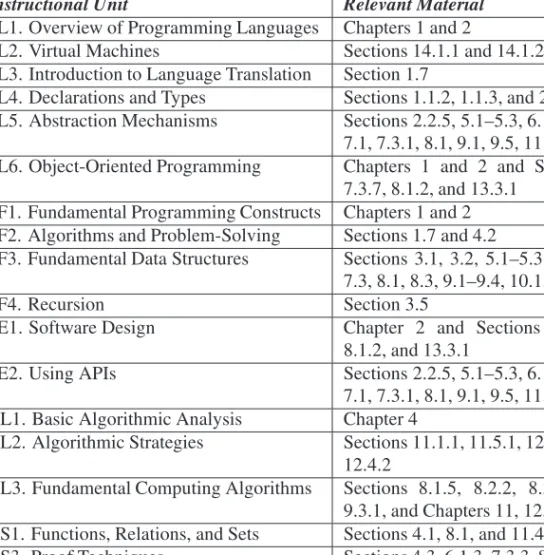
A C++ Primer
The chapters for this course are organized to provide a pedagogical path that begins with the basics of C++ programming and object-oriented design. We provide an early discussion of concrete structures, such as arrays and linked lists, in order to provide a concrete foundation to build upon when constructing other data structures.
Object-Oriented Design
We then add fundamental techniques such as recursion and algorithm analysis, and in the main part of the book introduce fundamental data structures and algorithms, concluding with a discussion of memory management (that is, the architectural underpinnings of data structures).
Trees
Heaps and Priority Queues
Sorting, Sets, and Selection
Strings and Dynamic Programming 13. Graph Algorithms
Memory Management and B-Trees A. Useful Mathematical Facts
Basic C++ Programming Elements
- A Simple C++ Program
- Fundamental Types
- Pointers, Arrays, and Structures
- Named Constants, Scope, and Namespaces
When this is done, the system allocator returns a reference to the first element of the array. So a local variable “hides” all global variables with the same name, as shown in the following example.
Expressions
- Changing Types through Casting
In C++, integer variables are 'signed' quantities by default, but they can be declared as 'unsigned', as in 'unsigned int x'. If the left operand of a right shift is unsigned, the shift is filled with zeros, otherwise the right shift is filled with the sign bit of the number (0 for positive numbers and 1 for negative numbers). To convert the value of the expression to type T we can use the notation “(T)exp.” to use. We call this a C-style cast.
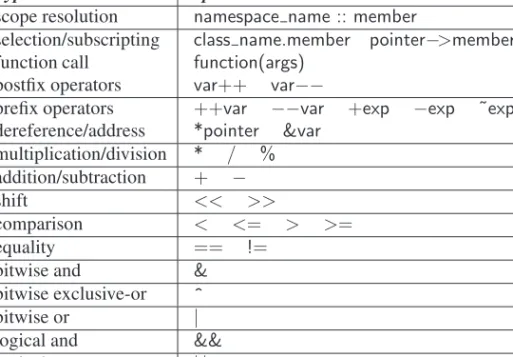
Control Flow
One loop tests a Boolean condition before executing an iteration of the loop body, and the other tests a condition afterward. Finally, the increment specifies what changes should be made at the end of each execution of the loop body.
Functions
- Argument Passing
- Overloading and Inlining
In the example above, the formal argument “n” is initialized to the actual value 6 when the function is called. This means that changes made to a formal argument in the function do not change the actual argument.
Classes
- Class Structure
- Constructors and Destructors
- Classes and Memory Allocation
- Class Friends and Class Members
- The Standard Template Library
In the above examples, we have shown member functions that are defined outside the body of the class. Because coord is a private member of Vector, members of the Matrix class would not have access to coord.
C++ Program and File Organization
- An Example Program
The main class structure is shown in the CreditCard.h header file and is shown in code snippet 1.2. Code snippet 1.3: The fileCreditCard.cpp, which contains the definition of the out-of-class member functions for classCreditCard. CREDIT CARD H has not yet been seen, so the header file is expanded by the preprocessor.
Therefore, any attempt to include the header file will find the CREDIT CARD Sy defined, so the file will not be expanded.
Writing a C++ Program
- Design
- Pseudo-Code
- Coding
- Testing and Debugging
We use indentation to indicate which actions should be included in the true actions and false actions. So good programmers must be mindful of their coding style and develop a style that communicates the important aspects of a program's design to both humans and computers. We should at least make sure that every method in the program is tested.
Even better, every code statement in the program must be executed at least once (statement coverage).
Exercises
R-1.20 Write a short C++ function that takes an integer n and returns the sum of all the integers less than n. R-1.21 Write a short C++ function that takes an integer n and returns the sum of all the odd integers less than n. C-1.4 Write a C++ function that takes an STLvectorofintvalues and prints all the odd values in the vector.
This means that each line is printed in the correct order, but the order of the lines is reversed.
Goals, Principles, and Patterns
- Object-Oriented Design Goals
- Object-Oriented Design Principles
- Design Patterns
This is the only part of the class that can be accessed by a user of the class. A class defines the data that is stored and the operations supported by objects that are instances of the class. One of the main advantages of encapsulation is that it gives the programmer freedom in implementing the details of a system.
One of the advantages of object-oriented design is that it facilitates reusable, robust, and adaptable software.
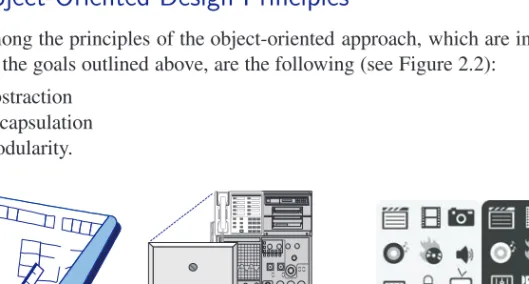
Inheritance and Polymorphism
- Inheritance in C++
- Polymorphism
- Examples of Inheritance in C++
- Multiple Inheritance and Class Casting
- Interfaces and Abstract Classes
For this reason, the constructor for a base class must be called in the initialization list (see Section 1.5.2) of the derived class. Protected and public members of the base class become protected and public members of the derived class, respectively. When a class implements an interface, it must implement all member functions declared in the interface.
Thus, each derived class must provide concrete definitions for all pure virtual functions of the base class.
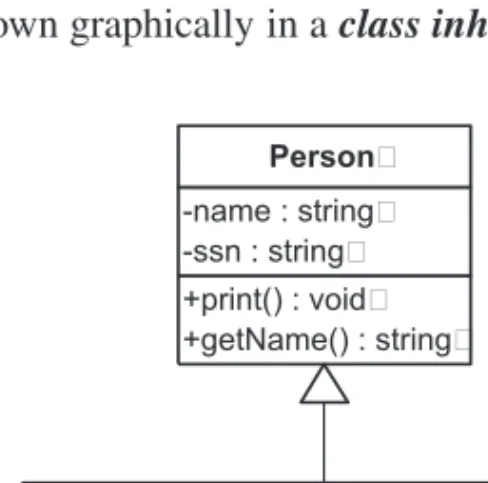
Templates
- Function Templates
- Class Templates
The compiler looks at the argument types and determines which form of the function to instantiate. We have omitted many of the other member functions, such as the copy constructor, the assignment operator, and the destructor. The template parameter T takes the place of the actual type that will be stored in the array.
To instantiate a concrete instance of the classBasicVector, we specify the class name followed by the actual type parameter enclosed in angle brackets (<..>).
Exceptions
- Exception Objects
- Throwing and Catching Exceptions
- Exception Specification
If an exception is thrown, then the control immediately jumps to the appropriate catch block for that exception. If, on the other hand, an exception is thrown, execution in the try block ends at that point and execution jumps to the first catch block that matches the exception thrown. For example, if NegativeRoot were thrown in the example above, it would be caught by the catch forMathException block.
When the execution of the catch block is complete, control flow continues with the first statement after the last catch block.
Exercises
R-2.18 Write a short C++ program that creates a Pair class that can store two objects declared as generic types. C-2.7 Write a program consisting of three classes, A, B, and C, such that B is a subclass of A and C is a subclass of B. C-2.9 Write a C++ program that can enter any polynomial in standard algebraic notation and outputs the first derivative of this polynomial.
Q-2.6 Write a C++ program that inputs a document and then outputs a bar graph of the frequencies of each alphabet character that appears in that document.
Using Arrays
- Storing Game Entries in an Array
- Sorting an Array
- Two-Dimensional Arrays and Positional Games
The approach is to shift all entries in the array whose scores are less than the scores of e to the right to make room for the new entry. If so, we check if the result of the last entry in the array (which is [maxEntries−1] entries) is at least as large as the result of e. To avoid overwriting existing array entries, we start at the right end of the array and work towards the left.
In this way, we continue with each element of the array, shifting it to the left until it is in the correct position.

Singly Linked Lists
- Implementing a Singly Linked List
- Insertion to the Front of a Singly Linked List
- Removal from the Front of a Singly Linked List
- Implementing a Generic Singly Linked List
Next, we consider how to remove an element from the front of a singly linked list. It's worth noting that we can't delete the last node of a singly linked list that easily, even if we had a pointer to it. The implementation of the singly linked list given in Section 3.2.1 assumes that the element type is a character string.
We can generate singly linked lists of different types by simply setting the template parameter as desired as shown in the following code fragment.
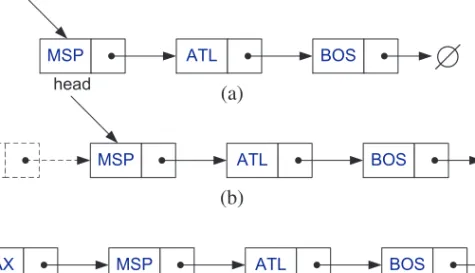
Doubly Linked Lists
- Insertion into a Doubly Linked List
- Removal from a Doubly Linked List
- A C++ Implementation
We declare DLinkedList to be a friend so that it can access the private members of the node. string typedef Elem; // element type list. classDNode { // doubly linked list node. Next, we present the definition of the doubly linked list class, DLinkedList, in Code Fragment 3.23. There are many other features we could have added to our simple implementation of a doubly linked list.
In a more robust implementation of a doubly linked list, we would design the member functions front, back, remove Front, and remove Back to throw an exception when attempting to perform any of these functions on an empty list.
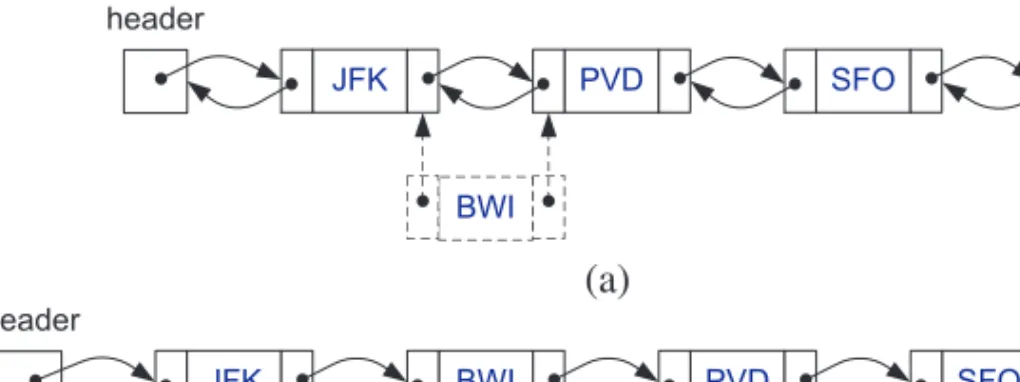
Circularly Linked Lists and List Reversal
- Circularly Linked Lists
- Reversing a Linked List
If this is the last node of the list (which can be tested by checking whether the node to be deleted is self-referential), we set the cursor to NULL. Fore, aft and forward we must first test whether the list is empty, otherwise the cursor pointer will be NULL. The first entry in the list is the element immediately following the cursor (which is where insertion and deletion occur), and the last entry in the list is the cursor (which is indicated by an asterisk).
Suppose we decide to replace “Stayin Alive” with “Disco Inferno.” We move the cursor twice so that “Stayin Alive” comes immediately after the cursor.
Recursion
- Linear Recursion
- Binary Recursion
- Multiple Recursion
Let's consider a C++ implementation of the factorial function shown in Code Fragment 3.36 under the name recursiveFactorial. Output: Return the elements in A starting at index i and ending at j if i< jthen. In Code Fragment 3.40, we give a nonrecursive algorithm that accomplishes this task by iterating through recursive calls to the algorithm of Code Fragment 3.39.
Output: Reversing the elements of A starts at index i and ends at j while i< jdo.
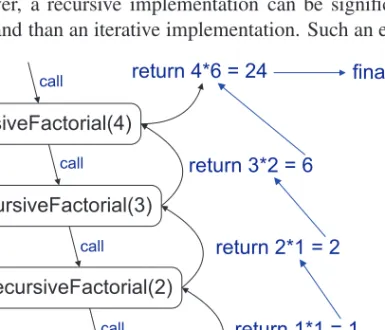
Exercises
R-3.15 Give a fully generic implementation of the circularly linked list data structure of Section 3.4.1 using a patterned class. C-3.7 Describe a fast recursive algorithm for returning a singly linked list L such that the order of the nodes is reversed from what it was before. C-3.11 Describe in detail an algorithm for returning a linked list L using only a constant amount of extra space and using no recursion.
P-3.5 Carry out the previous project, but use a linked list that is both circularly and doubly linked.
The Seven Functions Used in This Book
- The Constant Function
- The Logarithm Function
- The Linear Function
- The N-Log-N Function
- The Quadratic Function
- The Cubic Function and Other Polynomials
- The Exponential Function
- Comparing Growth Rates
Example 4.2: Below we demonstrate some interesting applications of the logarithm rules from Proposition 4.1 (using the usual convention that the base of a logarithm is 2 if omitted). In other words, this is the total number of operations performed by the nested loop if the number of operations performed inside the loop increases by one with each iteration of the outer loop. The integer d, which indicates the highest power of the polynomial, is called the degree of the polynomial.
To summarize, Table 4.1 shows each of the seven common functions used in algorithm analysis in order.

Analysis of Algorithms
- Experimental Studies
- Primitive Operations
- Asymptotic Notation
- Asymptotic Analysis
- Using the Big-Oh Notation
- A Recursive Algorithm for Computing Powers
This methodology aims to associate, with each algorithm, a function f(n) that characterizes the running time of the algorithm as a function of the input size n. Thus, we may wish to express the running time of an algorithm as a function of input size taken by averaging over all possible inputs of the same size. In the analysis of the algorithm, we focus on the rate of increase of the execution time as a function of the input size n, taking a "big picture" approach.
So, even when we use the big-O notation, we need to be at least somewhat mindful of the constant factors and lower order terms that we are "hiding".
