Chapter 2 introduces complexity analysis and, depending on your needs, some of the material in the chapter. Connect Four is a trademark of Milton Bradley Company in the United States and other countries.
Python Programming 101
Chapter Goals
Creating Objects
- Literal Values
- Non-literal Object Creation
In most cases, when an object is created, it is not created from a direct value. Of course, we need literal values in programming languages, but most of the time we already have an object and want to create another object using one or more existing objects.
Calling Methods on Objects
The variable is a reference to an object that is created using the object it refers to. Theother_object_value is a comma-separated sequence of references to other objects needed by the class or type to create an instance (i.e., an object) of that type.
Implementing a Class
- Creating Objects and Calling Methods
- The Dog Class
If a class had no accessor methods, we could put values into the object, but we could never retrieve them. The other arguments are passed by the programmer when the method is called (see the example of calling each method in Sect.1.4.1).
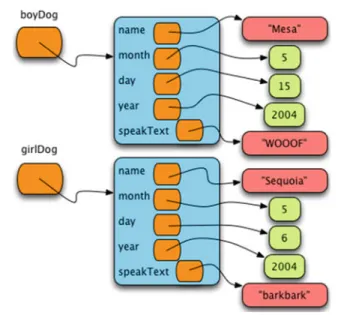
Operator Overloading
- The Dog Class with Overloaded Addition
The difference is that thestroperator must return a string suitable for human interaction, while the properator is called when an evaluable representation of the string is required. For example, if we wanted to define these two operators in the Dog class, the repr method would return the string "Dog('Mesa WOOOF')", while thestroperator could return only the name of the dog.
Importing Modules
Although this is not quite as convenient because you have to precede Turtle with "turtle", it is safe because the namespace of your module and the turtle module are kept separate. All identifiers in the turtle module are in the turtle namespace, while the local identifiers are in the local namespace.
Indentation in Python Programs
Which way you choose to import code may be a matter of personal preference, but there are some implications regarding using the appropriate method of importing code. In fact, there may be other identifiers that the turtle module determines that you are not aware of, which would also be identifiers that you should not use in your code.
The Main Function
- Python Program Structure
When a module is imported into another module, it will not perform its main function. Later, you'll have the option to write a module that you want to import into another module, so it's a good habit to always call the main function this way.
Reading from a File
- A Text File with Single Line Records
- Reading and Processing Single Line Records
- Pattern for Reading Single Line Records from a File
Since each record (that is, the drawing command) is on its own line in the file format described in Section 1.9.1, we can read the file by using the for loop to read the lines of the file. 4 # The body of the for loop is executed once for each line in the file.
Reading Multi-line Records from a File
- A Text File with Multiple Line Records
- Reading and Processing Multi-line Records
- Pattern for Reading Multi-line Records from a File
Notice that in line 13, the first line of the first record is read before the while loop. The first line of every other record is read at the end of the while loop on line 55.
A Container Class
Polymorphism
- Graphics Command Classes
The Accumulator Pattern
- List of Squares
- A Graphics Program
Implementing a GUI with Tkinter
- A GUI Drawing Application
The fill=tkinter.BOTH causes the button to 224 # expand to fill the entire width of the sidebar. 247 penColorButton = tkinter.Button(sideBar, text = "Pick Pen Color", command=getPenColor) 248 penColorButton.pack(fill=tkinter.BOTH).
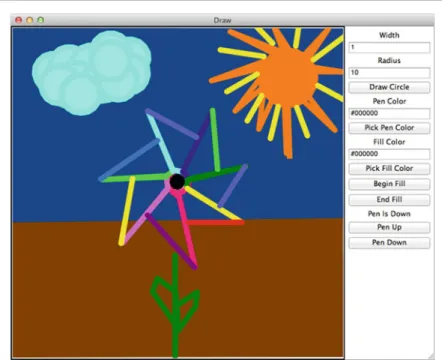
XML Files
- The Truck XML File
- The GoToCommand with XML Creation Code
- Writing Graphics Commands to an XML File
Apart from the XML format (ie the grammar), the content of an XML file is completely up to the programmer or programmers using the data. To write commands to a file, you can modify each command class to create an XML element when you convert it to a string using special__str__.
Reading XML Files
- Using an XML Parser
If we want to loop through all these elements, we can use a for loop as in the code in Section 1.16.1. In the code in section 1.16.1theattrvariable is a dictionary describing attribute names (i.e. keys) with their associated values.
Chapter Summary
Review Questions
What is an attribute in an XML document and how can you access the value of an attribute.
Programming Problems
You must ensure that your color string is 6 digits long and begins with a pound sign (i.e. #) to be a valid color string in Python.
Computational Complexity
Chapter Goals
Computer Architecture
- Running a Program
A much better analogy is a group of people, with each person representing a memory location within the computer's RAM. It takes exactly the same amount of time to store a value at any location within RAM.
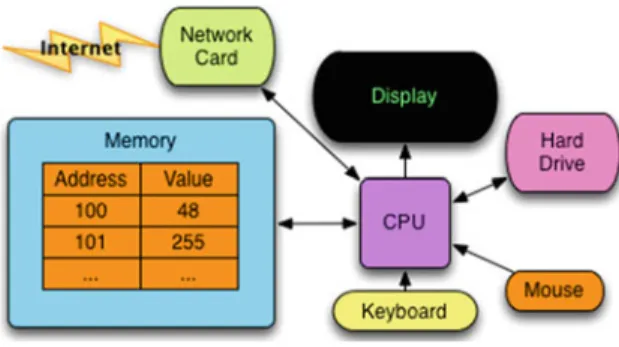
Accessing Elements in a Python List
- List Access Timing
- A Plot XML Sample
It is clear that the only trend is that the list size does not affect the average access time. Experimental data support the claim that list size does not affect average list access time.
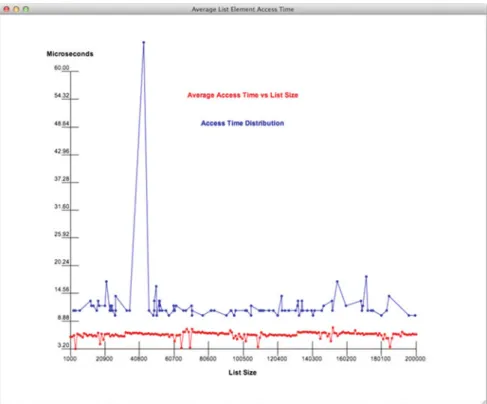
Big-Oh Notation
We have seen that the average time to access an element in a list is constant and does not depend on the list size. In the example in Fig.2.2, the list size is then in the definition and the average time to access an element in a list of size is the f(n).
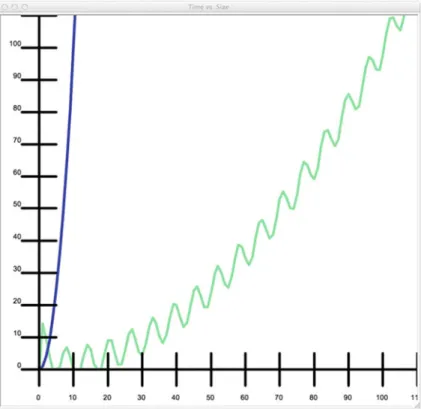
The PyList Append Operation
- Inefficient Append
The next time append is called, three elements must be used to form the new list. When the nth element is added to the sequence, ben elements will have to be copied to form the new list.
A Proof by Induction
Having proved that forn =2 holds, we can use the same formula to prove that the equality holds forn =3. As long as we have created this meta-test, we have proven that equality applies to everyone.
Making the PyList Append Efficient
- Efficient Append
To prove that the equality holds for n=2, we had to use the fact that the equality holds for n=1. The blue line in Figure 2.4 shows how PyList's add method works when the + operator is replaced by a call to the list's add method.
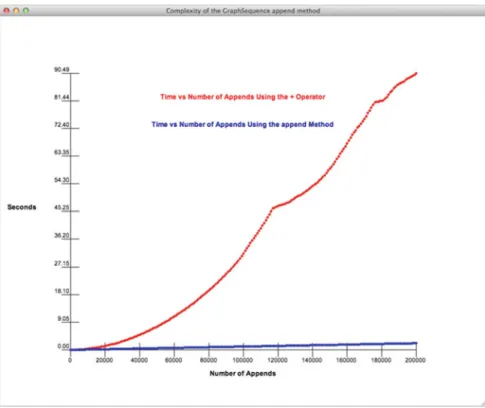
Commonly Occurring Computational Complexities
As each of these techniques are explored, you all also have the opportunity to write some fun programs and learn a lot about object-oriented programming.
More Asymptotic Notation
- Big-Oh Asymptotic Upper Bound
- Asymptotic Lower Bound
- Theta Asymptotic Tight Bound
But the blue line represents a strict bound on the complexity of the algorithm whose running time is depicted by the green line. Now, instead of saying that n-squared is an upper bound on the algorithm's behavior, we can proclaim that the algorithm actually runs in time proportional to n-squared.
Amortized Complexity
- A PyList Class
- Proof of Append Complexity
Our PyList append operation, when it runs out of space in the fixed-size list, will double the size of the list by copying all items from the old list to the new list as shown in the code in Section 2.10.1. Whenever the list runs out of space, a new list is allocated and all the old elements are copied to the new list.
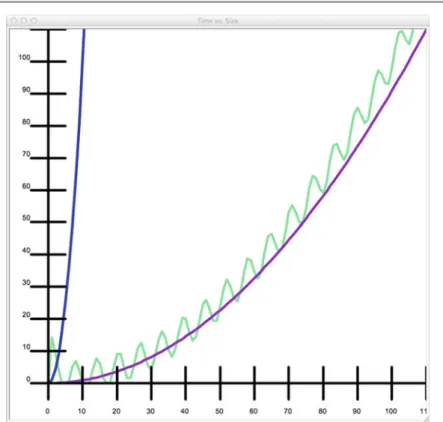
Chapter Summary
At this point, three cyber dollars are saved, which will be used in doubling the size of the list to four locations. When the list of size four fills up, two additional addition operations have occurred, storing five cyber dollars.
Review Questions
Programming Problems
The Clearable object must always keep track of the number of values currently stored in the object. Create one sequence for each different starting size of the Clearable data type and write your results in the plot format described in this chapter.
Recursion
Chapter Goals
You should also be able to write some simple recursive functions yourself without thinking too much about how they work. Additionally, you should be able to use a debugger to examine the contents of the runtime stack for a recursive function.
Scope
- Local Scope
- Enclosing Scope
- Global Scope
- Built-In Scope
- LEGB
During the execution of line 23 of the program in Figure 3.2, the identifier is valid in the local scope. During the execution of line 23 of the program in Figure 3.2, theval in the local scope is visible, but theval in the scope it covers is hidden.
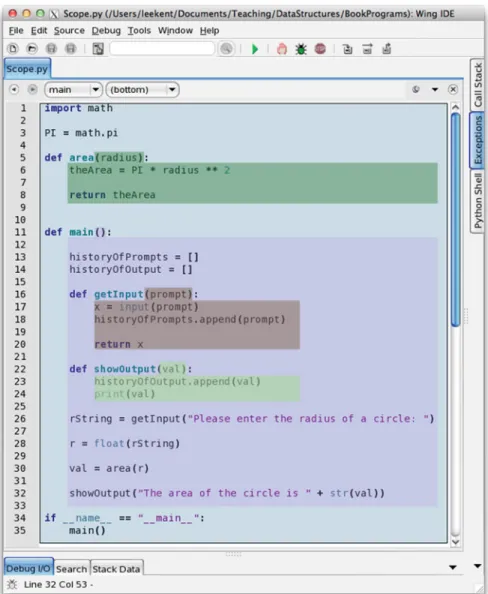
The Run-Time Stack and the Heap
When the interpreter returned from the function call, the corresponding activation record was pushed from the execution stack. The activation record for the showOutpoutfunction call was pushed onto the runtime stack when showOutput was called.
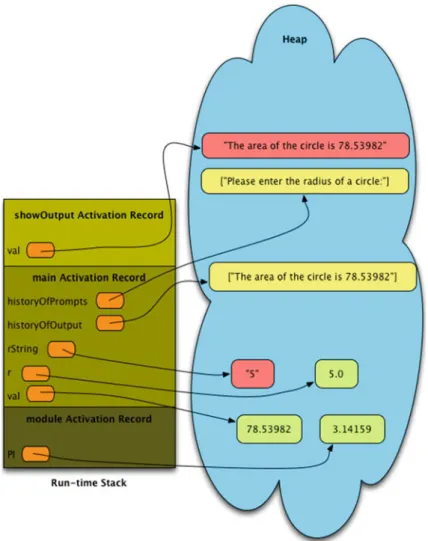
Writing a Recursive Function
- Sum of Integers
- Recursive Sum of Integers
- No Else Needed
The basis of a recursive function must be written first before the function is called recursively. The base case is a statement that handles a very simple case in the recursive function by returning a value.
Tracing the Execution of a Recursive Function
The activation record for the function call when it was 0 is retrieved from the runtime stack. When the function returns, the activation record space is reclaimed for later use.
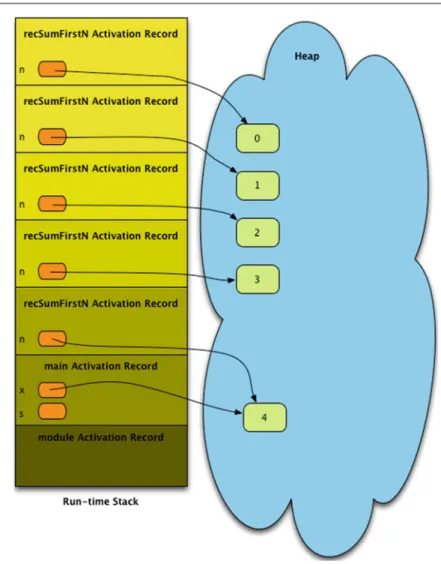
Recursion in Computer Graphics
- Recursive Spiral
It has a basic example that is written first: when the length of the side is zero, it exits. There is some slicing going on to convert the color string from a hexadecimal string to an integer so that 1024, modulo 2, can be added to 24.
Recursion on Lists and Strings
- List Recursion
- Reversing a List
- Reversing a String
- Another Version of Reverse
For example, this example could be rewritten so that the index grows to the length of the list. In that case, the distance between the index and the length of the list is the value that would be decremented on each recursive call.
Using Type Reflection
- Reflection Reverse
It's important to note that you don't need to physically make a list or array smaller to use it in a recursive function. As long as indexing is available to you, a recursive function can use an index into a list or array, and the index can get smaller with each recursive call.
Chapter Summary
Review Questions
Programming Problems
Place this function in a program that draws at least one tree. HINT: In your drawBranch function, after you've drawn the branch (and all of its sub-branches), you want to return the turtle to the original position and direction where you started. Polar coordinates are a way of specifying any point in the plane with an angle and a radius.
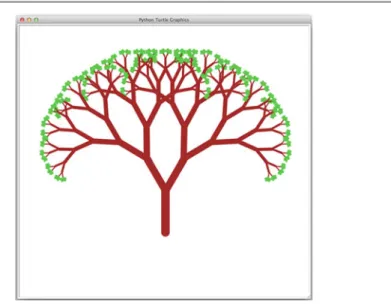
Sequences
Chapter Goals
What is the complexity of many of the common operations on series, and how is that complexity affected by the underlying organization of the data.
Lists
- The PyList Datatype
- The PyList Constructor
- PyList Get and Set
- PyList Concatenate
- PyList Append
- PyList Insert
- PyList Delete
- PyList Equality Test
- PyList Iteration
- PyList Length
- PyList Membership
- PyList String Conversion
- PyList String Representation
All the locations used in the internal list will appear at the beginning of the list. Testing for membership in a list means checking whether an element is one of the items in the list.
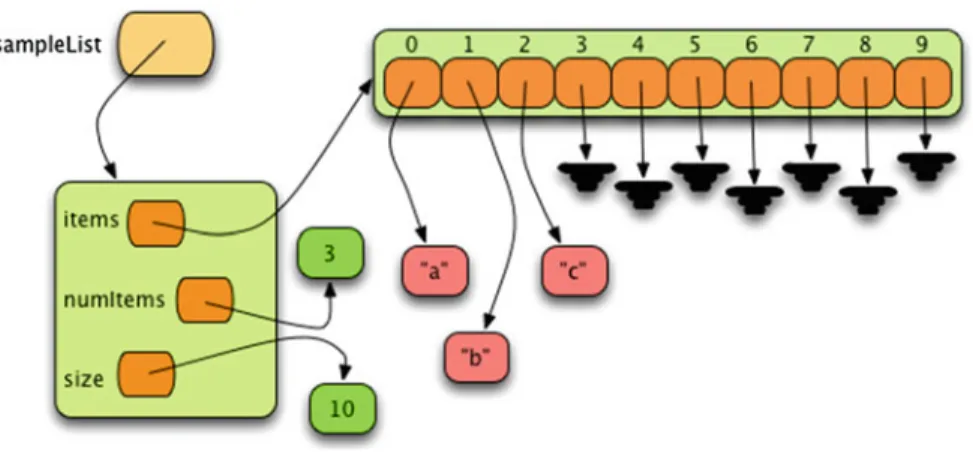
Cloning Objects
Python includes a function called eval that takes a string containing an expression and evaluates the expression in the string. In the case of the PyList class, the string format would be something like "PyList([1,2,3])" for the sequence of PyLists containing these elements.
Item Ordering
- The Point Class
- Calling the Sort Method
Comparing lst and lst2 did not work because the items in the two lists are not orderable. Since there is no natural order, there seems no reason to sort them.
Selection Sort
- Selection Sort’s Select Function
- The Selection Sort Code
Since the smallest value is now in the first location in the sequence, the selection sort algorithm starts looking for the smallest value from the second position in the list. Then the selection sort algorithm searches for the smallest item starting from the third place in the sequence.
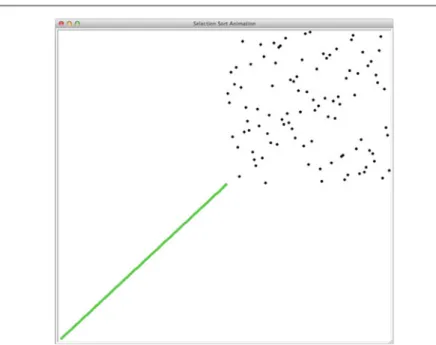
Merge Sort
- The Merge Sort Code
This analysis seems to suggest that the complexity of the merge sort algorithm is O(n2) because there are approximate merges, each of which is only O(n). In the second version of the list, two joins are performed for lists of length two.
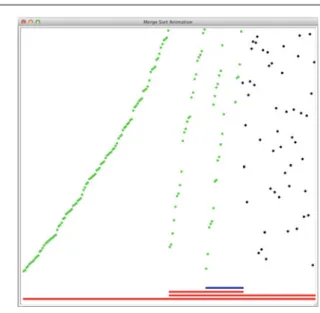
Quicksort
- The Quicksort Code
110 4 Pivot Sequences is that the quicksort algorithm starts by randomizing the sequence. All values above the axis will end up in the division to the right of the axis, and all values to the left of the axis are less than the axis.
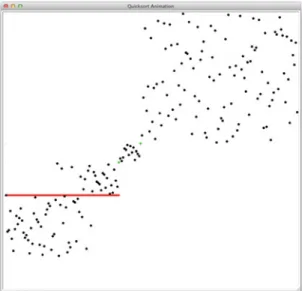
Two-Dimensional Sequences
- The Board Class
- The X, O, and Dummy Classes
Since each row is itself a list in the Board class, we can just use the built-in class class for the rows of the array. Then 21 # calling __init__ on the superclass initializes the part of the object that is 22 # a RawTurtle.
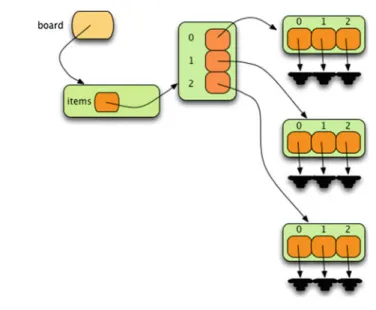
The Minimax Algorithm
To deal with this, we can have the code that executes queue the computer to do some of the work. It should be noted that tic tac toe has a small enough search space that the computer can solve the game.
Linked Lists
- The Node Class
- The LinkedList Constructor
- LinkedList Get and Set
- LinkedList Concatenate
- LinkedList Append
- LinkedList Insert
- Other Linked List Operations
We keep a reference to the first node in the sequence so that we can traverse nodes if necessary. Changing the next cursor node pointer to point to the node after "b".
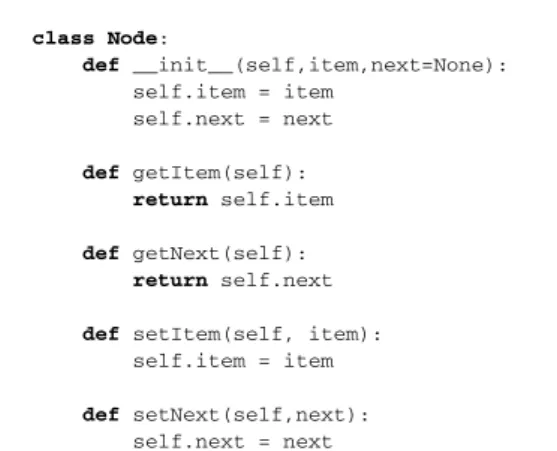
Stacks and Queues
- The Stack Class Code
- Infix Expression Evaluation
- The Operate Procedure
- Example
- Radix Sort
- The CharAt Function
- Radix Sort Example
If the given operator is a left socket, we push it onto the operator stack and return. This forces 9 to be subtracted from 44 leaving 35 on the operand stack and the operator stack empty.
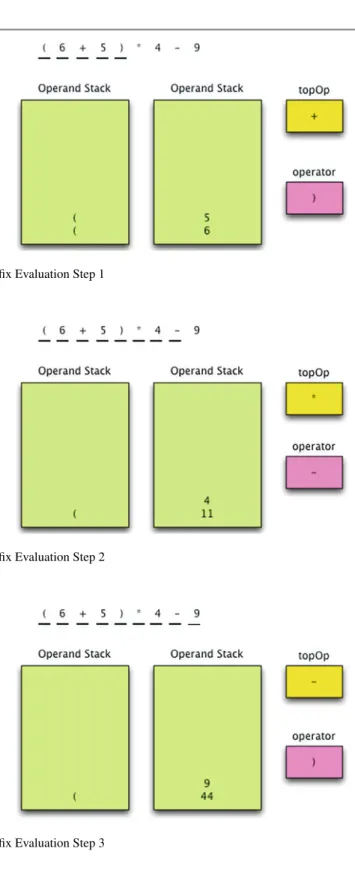
Chapter Summary
Review Questions
Under what circumstances could merge sort perform better than quicksort. What is the purpose of the start parameter for the selection function of the selection sort algorithm.
Programming Problems
The binary search algorithm starts by looking for the item in the middle of the sequence. If it is not found there, because the list is sorted, the binary search algorithm knows whether to search the left or right side of the sequence.
Sets and Maps
Chapter Goals
Playing Sudoku
Applying rules like this reduces the number of possible values for each cell in the puzzle. The second line looks at each cell within a group and discards all items found in other cells in the group.
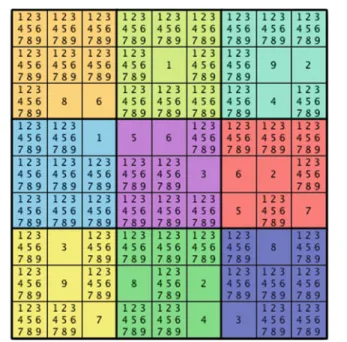
Sets
Applying this rule to the fifth row in Figure 5-3 reduces the fourth column to a 1, because 1 does not appear in any other cell in the fifth row. How to calculate the union of two sets in O(n) time if we want to make sure that there are no duplicates in the set.
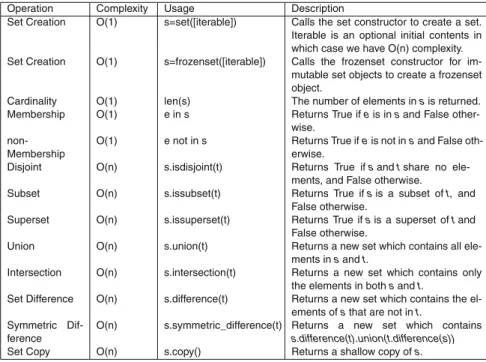
Hashing
These zeros and ones can be interpreted however we like, including as an index into a list. Obviously, some work needs to be done to turn this integer hash into an acceptable index into a list.
The HashSet Class
- The HashSet Constructor
- Storing an Item
- Collision Resolution
- HashSet Add Helper Function
- The Load Factor
- HashSet Add
- Deleting an Item
- HashSet Remove Helper Function
- HashSet Remove
- Finding an Item
- HashSet Membership
- Iterating Over a Set
- Other Set Operations
- HashSet Difference Update
- HashSet Difference
The code in Section 5.5.4 does not add an item that is already in the list. In the hashset implementation, we chose to double the size of the list when rehashing was necessary.
Solving Sudoku
- The Sudoku Reduce Function
We are guaranteed that it will terminate as each iteration of the algorithm reduces the number of items in some of the sets of the puzzle. ThereduceGroupsfunction above will presumably call reduceGroup on each of the groups in its list and it will return True if any of the groups have been reduced and False otherwise.
Maps
- The HashMap Class
- HashSet Get Item
- The HashMap Class
A __eq__method must be defined in the __KVPairclass definition so that the keys are compared when comparing two elements in the hash map. The __hash____KVPair method only hashes the key value, since the keys are used to find key/value pairs in the hash map.
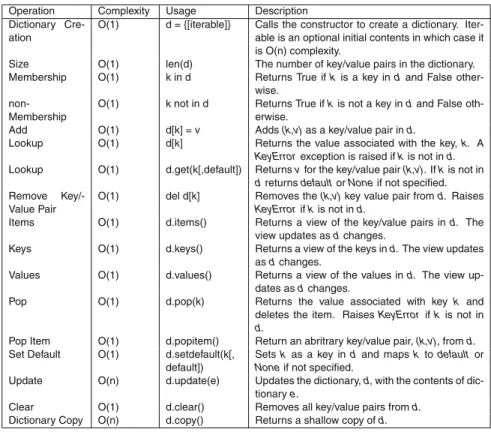
Memoization
- A Memoized Fibonacci Function
As you can see from Fig.5.7, it takes many calls to the fib function to calculate fib(5). It took 15 calls to fib to calculate fib(5) and from the figure we can see that it takes 9 calls to calculate fib(4).
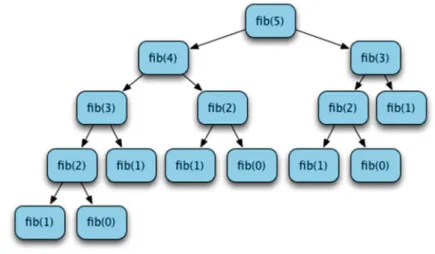
Correlating Two Sources of Information
For example, in the tic tac toe problem of Chapter 4, the minimax function is called on many tables that are identical. The minimax function does not care if an X is placed in the upper right corner first followed by the lower left corner or vice versa.
Chapter Summary
Review Questions
Programming Problems
Save the class in a file called hashset.py so that it can be imported into other programs. In other words, the X's, O's and Dummy objects must account for the hash value of the board so that each board has its own unique hash value.
Trees
Chapter Goals
What is depth first search and how does it relate to trees and search problems.
Abstract Syntax Trees and Expressions
- Constructing ASTs
To represent this in the computer, we could define a class for each type of node. So we can evaluate the abstract syntax tree, each node in the tree will have an eval method defined on it.
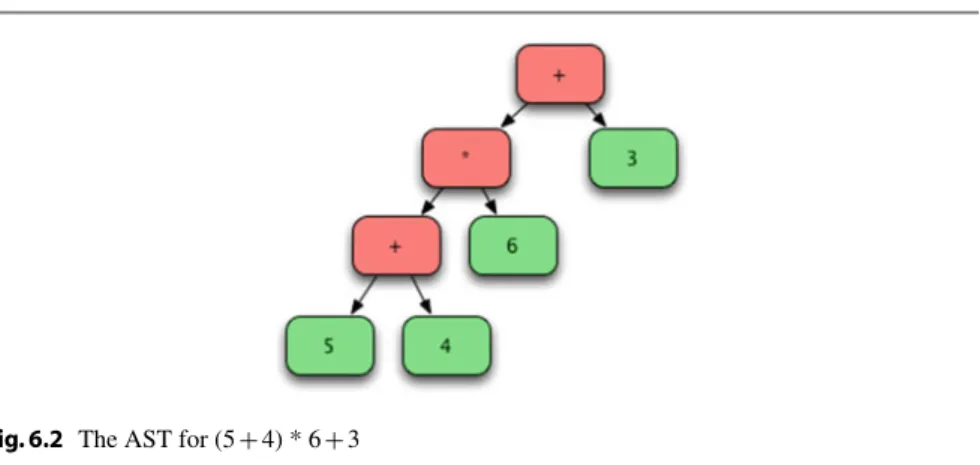
Prefix and Postfix Expressions
- AST Tree Traversal
Note that because of the way a mail order traversal is written, parentheses are never needed in postfix expressions. In a preorder traversal, each binary operator is added to the string before the two operands.
Parsing Prefix Expressions
- The Prefix Expression Grammar G = (N , T , P , E ) where
- A Prefix Expression Parser
- The Postfix Expression Grammar G = (N , T , P ,E) where
The inorder methods in Sect.6.3.1 provide an inorder traversal because each binary operator is added to the stringin between the two operands. A function, like the E function in Sect.6.4.2, that reads tokens and returns an abstract syntax tree is called aparser.
Binary Search Trees
- The BinarySearchTree Class
Because that subtree contains 2, the 1 is inserted into the left subtree of the node containing 2. The last part of the program in Sect.6.5.1 iterates over the tree in the main function.
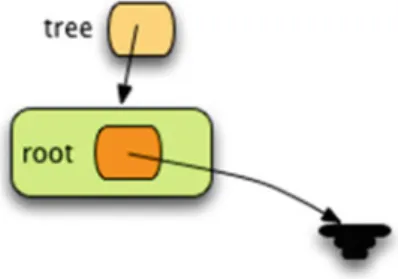
Search Spaces
- Depth-First Search Algorithm
- Sudoku Depth-First Search
- Calling Sudoku’s Solve Function
Deletion from a binary search tree can also be done in O(log n) time in the average case. Looking up a value in a binary search tree can also be done in O(log n) time in the average case.
Chapter Summary
Review Questions
Programming Problems
Then you use this function to get the rightmost value of the left subtree of the node to delete. In Fig.6.15, the 5 is eliminated by placing the node containing 5 in 4, the rightmost value of the left subtree.
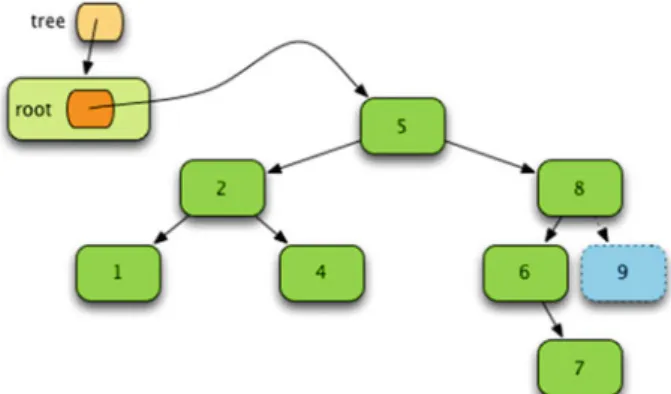
Chapter Goals
Many problems in computer science and mathematics can be reduced to a series of states and a series of transitions between these states. Many of the algorithms in graph theory are named after the mathematician who developed or discovered them.
Graph Notation
Both directed and undirected graphs can be used to model many different types of problems. A weighted graph can be used to represent the state of many different types of problems.
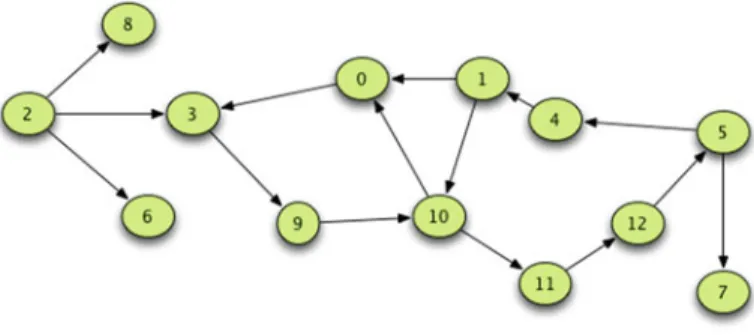
Searching a Graph