But this book is not designed to cover everything, and I recommend reading other books and the Python documentation to fill in the gaps. In terms of structuring a course around this book or the lesson itself, the foundation is most of Part I.
Installing Python
IDLE
HOW TO GET STARTED either do that or start IDLE and open the file through the File menu. Keyboard Shortcuts The following keystrokes work in IDLE and can really speed up your work.
A first program
CTRL+Z Undo the last keystroke or group of keystrokes CTRL+SHIFT+Z Redo the last keystroke or group of keystrokes.
Typing things in
Getting input
Printing
Variables
In the example below, we perform a calculation and need to use the result of the calculation in several places in the program. If we store the result of the calculation in a variable, we only have to do the calculation once.
Exercises
The fourth line is not indented, so it is not part of the loop and is executed only once, after the loop has completed. The way the loop works is we print a C, then a D, then loop back to the beginning of the loop and print a C and another D, etc.
The loop variable
The range function
FOR LOOPS If we want the list of values to start with a value other than 0, we can do so by specifying the start value. Another thing we can do is to increment the list of values by more than one at a time.
A Trickier Example
This leads to one peculiarity of the scope function - it stops one less where we think it should. If you wanted the list to contain the numbers 1 to 5 (5 inclusive), you should use dorange(1,6).
Exercises
Write a program that asks the user how many Fibonacci numbers to print and then prints that many. Because of the way computer chips are designed, whole numbers and decimal numbers are represented differently in computers.
Math Operators
It would be nice if there were no precision limits, but the calculations run much faster if you cut off the numbers at some point. If you need to "turn around" and go back to the beginning, the modulo is useful.
Order of operations
Random numbers
Math functions
Built-in math functions There are two built-in math functions, abs (absolute value) and around which are available without importing theme module. The round function takes two arguments: the first is the number to be rounded and the second is the number of decimal places to round to.
Getting help from Python
Using the Shell as a Calculator
Exercises
Write a program that prompts the user for a number and prints the factorial of that number. Write a program that asks the user to enter a year and prints the date of Easter in that year.
Conditional operators
Common Mistakes
That is, if the score is a 95, the first program will print an A, but then go on and check if the score is a B, C, etc., which is a bit of a waste. Brugelif, as soon as we find out where the result matches, we stop checking the conditions and jump all the way to the end of the entire block of statements.
Exercises
Write a program that asks the user how many items they are buying and prints the total cost. Write a program that prompts the user for a year and prints whether it is a leap year or not.
Counting
We set it to 0 to indicate that no numbers greater than 10 were found at the start of the program. Example 2 This modification of the previous example counts how many of the numbers the user enters are greater than 10 and also how many are equal to 0.
Summing
A few notes here: First, because of the quirk of the range function mentioned earlier, we need to use range(1,101) to loop through the numbers 1 through 100. Next, to check if a number ends in 4, a fun math trick is to check if there's a remainder of 4 left when you divide by 10.
Swapping
Flag variables
Maxes and mins
Comments
Simple debugging
MISCELLANEOUS TOPICS In a condition for an if statement, you can put a print statement inside the if block to see if the condition is triggered.
Example programs
The reason for this is that by indenting the print statement, we have made it part of the for loop, so the print statement will be executed 10,000 times. Now, not only is it part of the for loop, but it's also part of the if statement.
Exercises
Write a program that prompts the user to enter a number and prints the sum of the divisors of that number. At the end of the program, print a message that varies depending on how many questions the player got right.
Concatenation and repetition
The in operator
Indexing
Slices
If we leave the start location blank, it defaults to the beginning of the string. If we leave the end location blank, it defaults to the end of the string.
Changing individual characters of a string
In the example above, s[2:5] e.g. the characters at indices 2, 3 and 4, but not the character at index 5. The most useful step is -1, which loops backwards through the string and returns the order of the characters.
Looping
Slices have the same property as the range function in that they do not include the end location.
String methods
A note about index If you try to find the index of something that is not in a string, Python will throw an error.
Escape characters
Examples
We want to push one character of the name the first time through the loop, two characters the second time, etc. Example 6 Write a program that, given a string containing a decimal number, prints the decimal part of the number.
Exercises
Write a program that prompts the user to enter a word and prints whether that word contains any vowels. Write a program that prompts the user to enter a word and then capitalize every other letter in that word.
Similarities to strings
Built-in functions
List methods
Miscellaneous
Examples
If we decide to change one of the questions or the order of the questions, then there is a fair amount of rewriting. If we decide to change the game design, like not showing the user the correct answer, then every single block of code has to be rewritten.
Exercises
Mof the same size and adds their elements together to form a new list whose elements are the sums of the corresponding elements in L and M. Write a program that prompts the user for an integer and creates a list consisting of factors of that integer. The way it works is to shift each character in the message by a random amount between 1 and 26 characters, wrapping around the alphabet if necessary.
The first two names on the shuffled list become the first team, the next two names become the second team, and so on. Note that we use the optional third argument of torange to skip the list of names by two.
What we need to do is convert our string to a list, apply random to it, and then convert the list back to a string. To turn a string into a list, we can use list(s). See Section 10.1.) To convert a list back to a string, we'll use concatenation.
List comprehensions
Using list comprehensions
Two-dimensional lists
Note You can certainly get away without using list comprehensions, but once you get the hang of them, you'll find that they're both faster to write and easier to read than the longer ways of creating lists.
Exercises
Your program should then choose a random location in the list that contains a zero and turn it into a one. Another way is shown below on the right. a) Write code that translates from the left view to the right.
Examples
In the code below, the while loop works much the same as the if statement in the previous example. The condition on the while loop guarantees that we don't get to the print job until the user enters a valid temperature.
Infinite loops
It checks to see if it is less than 50, and since it is 2, which is less than 50, the entered code must be executed again. At this point, the while condition will finally no longer be true, and the program jumps to the first statement after thewhile, which printsBye!.
The break statement
So we print one more time, add 2, and then go back and check the while loop condition again.
The else statement
If we get all the way through the loop without finding a divisor, nine will equal , in which case the number must be prime. If we get all the way through the loop without breaking, then we haven't found a divisor.
The guessing game, more nicely done
Since this comparison will be done repeatedly and depends on the user's guesses, it is placed in the loop after the input statement. When they run out of turns, the while loop stops looping and program control shifts to anything outside the loop.
Exercises
The program must continue until the player guesses all the letters of the word or gets five letters wrong. The program should now display the grid with the previous character and the new character displayed.
Booleans
Shortcuts
Short-circuiting
Continuation
String formatting
Nested loops
Here is a program that finds all Pythagorean triples(x,y,z)wherex,y,andare positive and less than 100. To get rid of these redundancies, change the second loop to run from x to 100.
Exercises
Write a program that repeatedly asks the user to enter a football score in win-loss format (eg 27-13 or 21-3). Write a program that repeatedly asks the user to enter their birthday in month/day format (eg 12/25 or 2/14).
Dictionary examples
Empty dictionary The empty dictionary is {}, which is the dictionary equivalent of [] for lists or ''for strings. Important note The order of items in a dictionary will not necessarily be the order in which they are placed in the dictionary.
Working with dictionaries
List of keys and values The following table illustrates the ways to get lists of keys and values from a dictionary. The following simple example creates a dictionary from a list of words, where the values are the lengths of the words:.
Counting words
To get to the individual words, we'll use the split method to convert the string into a list of individual words. When we sort a list of tulips, the sorting is done by the first entry, which in this case is a word.
Exercises
Write a program that prompts the user for the key and type of the chord and outputs the notes of the chord. One way to solve this problem is to create a dictionary whose keys are indices into the user string of non-star characters and whose values are those characters.
Writing to files
Examples
Wordplay
TEXT LISTS Assuming the wordlist file is wordlist.txt, we can load the words into a list using the line below. EXERCISES 113 Note that this is not a very efficient way of doing things since we need to scan most of the list.
Exercises
How many words contain at least one of the letters r, s, t, l, n, e. d) The percentage of words containing at least one of the letters r, s, t, l, n, e (e) All words without vowels. f) All words containing every vowel. G). TEXT FILES (x) All words of the formabcd*dcba, where * is any long sequence of letters.
Arguments
Put the code in a function and when you need a box, just call the function instead of writing several lines of redundant code. An advantage of this is that if you decide to change the size of the box, you only need to change the code in the function, whereas if you had copied and pasted the box drawing code everywhere you needed it, you would have to change all of them.
Returning values
The same effect can be achieved with an if/else statement, but in some cases, using returnn can make your code simpler and more readable.
Default arguments and keyword arguments
Local variables
If a function wants to change the value of this variable, we must tell it that time_lefti is a global variable. On the other hand, if we just want to use the value of a global variable, we don't need aglobalstatement.
Exercises
On the other hand, etc. before the middle entry comes, then search the first half of the list. If it comes to the middle entry, then look for the second half of the list.
Creating your own classes
In some cases that is useful, but you have to be careful with it. In Python, all variables are public and it is up to the programmer to be responsible for them.
Inheritance
A playing-card example
The idea here is that Card_group represents an arbitrary group of cards, and Standard_deck represents a specific group of cards, namely the standard deck of 52 cards used in most card games. Suppose we've just created a single class that represents a standard deck along with all the common operations like shuffling.
A Tic-tac-toe example
A simple computer player would call that method and userrandom.choicemethod to choose a random element from the returned list of spots.
Further topics
Exercises
The class should have a list called old_passwords that contains all of the user's previous passwords. The user of the class must pass the list of words they want to use to the class.
Labels
Entry boxes
Buttons
When the button is clicked, the callback function is called, which changes the label to say Button clicked. We only use one callback function and it has one argument, which indicates which button was clicked.
Global variables
We don't really care what those things are because they will be replaced with buttons. But in the short programs we're going to write, we should be okay.
Tic-tac-toe
In this example, we'll create a frame to group all the letter buttons into one big widget. Then, for all the widgets we want to include in the framework, we include the name of the framework as the first argument in the widget declaration.
Colors
The framework's job is to hold other widgets and basically combine them into one big widget. We still need to grid the widgets, but now the rows and columns will be relative to the frame.
Images
File Types One unfortunate limitation of Tkinter is the only common image file type it can use is GIF. If you want to use other types of files, one solution is to use the Python Imaging Library, which will be covered in Section 18.2.
Canvases
Check buttons and Radio buttons
This variable, show_totals, will be 0 when the control button is not selected and 1 when it is checked. Commands Both control buttons and radio buttons have a command, where you can set a callback function to run whenever the button is selected or selected.
Text widget
GUI PROGRAMMING II The one thing to note here is that we need to bind the check button to a variable, and it can't be just any variable, it needs to be a special kind of Tkinter variable called anIntVar. The value of the IntVarobjectcolor will be 1, 2, or 3 depending on whether the left, middle, or right button is selected.
Scale widget
GUI Events
You can use the program from earlier in this section to find the names of all the keys. You can also capture key combinations, such as
Event examples
First, every time the mouse is moved across the canvas, the mouse_motion_event function is called. Dragging is usually done in the b1_motion_event function, which is called when the left mouse button is pressed and the mouse is moved.
Disabling things
Getting the state of a widget
GUI PROGRAMMING III This can be used with buttons, canvases, etc, and it can be used with any of their properties such as bg, fg, state, etc.
Message boxes
Destroying things
Updating
GUI PROGRAMMING III In that function, if you want to change something on the screen, pause for a short while, and then change something else, you need to tell Tkinter to update the screen before the pause. If you just want to update a particular widget and nothing else, you can use that widget's update method.
Dialogs
The return value ofaskopenfilenames is a list of files, which is empty if no file is selected. A brief example Here is an example that opens a file dialog that allows you to select a text file.
Menu bars
New windows
StringVar
More with GUIs
The currentprint function also has those useful optional arguments, sepanend, which are not available in Python 2. When you use formatting codes in curly braces, you must specify an argument number in Python 2.
The Python Imaging Library
We also use the conversion method to convert the image to RGB (Red-Green-Blue) format. The Image object has a method called load that provides access to the individual pixels that make up the image.
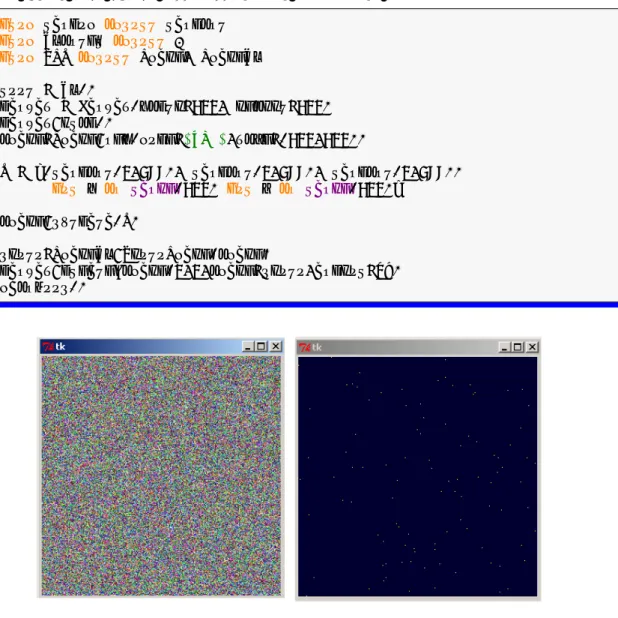
Pygame
The draw.rectangle method works in the same way as the canvass_create_rectangle method, with the exception of some bracket differences. A nice feature of it is that we can pass a list of points instead of having to plot each thing in the list individually.
Mutability and References
When we set = 'Hello', the string object Hello is somewhere in memory and is a reference to it. Then when we do L[0]=9, we just change the thing that points to, and still copy points to[1,2,3].
Tuples
Sets
Remember that curly braces are also used to denote dictionaries, and {} is the empty dictionary. Note that Python will store the data in a set in any order it wants, not necessarily the order you specify.
Unicode
More with strings
Miscellaneous tips and tricks
Running your Python programs on other computers
Dates and times
Working with files and directories
Running and quitting programs
Zip files
Getting files from the internet
Sound
Your own modules
Syntax
Summary
Groups
Other functions
Examples
Scientific notation
Comparing floating point numbers
Fractions
The decimal module
Complex numbers
More with lists and arrays
Random numbers
Miscellaneous topics
Using the Python shell as a calculator
Anonymous functions
Recursion
The operator module
More about function arguments
Cartesian product
Grouping things
Miscellaneous things from itertools
Counting things
Try/except/else
More with exceptions