The purpose of this book is not primarily to teach Raku, but to learn the art of programming using the Raku language. Uceta translated this book into Spanish and discovered quite a few typos in the process, which he corrected in the Github repository.
Starting with the Basics
The main reason for that is that I wanted to make a distinction between, on the one hand, relatively basic concepts that are really necessary for any programmer who uses Raku, and, on the other hand, more advanced concepts that a good programmer should know but may be needed less often in day-to-day development work. The second part focuses on various programming paradigms and more advanced programming techniques that I believe are of paramount importance, but should probably be studied in the context of a second, more advanced semester.
The Way of the Program
- What is a Program?
- Running Raku
- The First Program 7
- The First Program
- Arithmetic Operators
- Values and Types
- Values and Types 9 These values belong to different types: 2 is an integer, 40 + 2 is also an integer, 84/2
- Formal and Natural Languages 11
- Formal and Natural Languages
- Debugging
- Glossary
- Exercises 13 Compiler A program that reads another program and transforms it into executable com-CompilerA program that reads another program and transforms it into executable com-
- Exercises
For example, in "Hello, World!" program, what happens if you omit one of the quotation marks. If you try to print a string, what happens if you omit one or both of the quotes.
Variables, Expressions and Statements
- Assignment Statements
- Variable Names
- Variable Names 17
- Expressions and Statements
- Expressions and Statements 19 The first line is an assignment statement that gives a value to $n. The second line is a print
- Script Mode
- One-Liner Mode
- Order of Operations
- String Operations 23
- String Operations
- Comments
- Debugging
- Glossary 25 Runtime error The second type of error is a runtime error, so called because the error doesRuntime errorThe second type of error is a runtime error, so called because the error does
- Glossary
- Exercises
When you type an expression at the prompt, the interpreter evaluates it, which means it finds the value of the expression. The terms on either side of the operator (here 17 and 25) are sometimes called the operands of the operation (in this case an addition).
Functions
Function Calls
Note that in Raku many built-in functions can also use amethod call syntax with so-called dot notation.
Function Calls 29 We’ll come back to methods in the next section
Functions and Methods
Math functions
Math functions 31
Composition
In the first string, $vari within the string is simply replaced by its value 42 because the string is enclosed in double quotes;. There are other quote constructs that offer finer control over how variables and special characters appear in the output, but single and double quotes are the most useful.
Definitions and Uses
Flow of Execution
Parameters and Arguments
When the above subroutine is called, it prints the contents of the parameter (whatever it is) twice. The name of the variable we pass as an argument ($declaration) has nothing to do with the name of the parameter ($value).
Variables and Parameters Are Local 37
Variables and Parameters Are Local
Stack Diagrams
Fruitful Functions and Void Functions
Fruitful Functions and Void Functions 39 But in a script, if you call a fruitful function all by itself, the return value is lost forever! In
Function Signatures
Immutable and Mutable Parameters
Functions and Subroutines as First-Class Citizens
Functions and Subroutines as First-Class Citizens 43 my $greet = sub {
Why Functions and Subroutines?
Debugging
Glossary
Stack diagram A graphical representation of a collection of subroutines, their variables, and the values they refer to. Function signature The part of a function's definition (usually between parentheses) that defines its parameters and possibly their types and other properties.
Exercises
First-class object Raku's subroutines would be higher-order objects or first-class objects because they can be passed as arguments from other subroutines or return values just like any other object.
Exercises 47 1. Type this example into a script and test it
Loops, Conditionals, and Recursion
- Integer Division and Modulo
- Boolean Expressions
- Boolean Expressions 51
- Logical Operators
- Logical Operators 53 with some other operators, they have a higher priority of execution. We will come back to
- Conditional Execution
- Alternative Execution
- Chained Conditionals
- Nested Conditionals
- If Conditionals as Statement Modifiers
- Unless Conditional Statement
- For Loops
- Recursion
- Stack Diagrams for Recursive Subroutines 61
- Stack Diagrams for Recursive Subroutines
- Infinite Recursion
- Keyboard Input
- Program Arguments and the MAIN Subroutine
- Debugging 63
- Debugging
- Glossary
- Exercises
Suppose you need to calculate and print the product of the first five positive digits (1 to 5). Flow of execution immediately returns to the caller and the remaining lines of the subroutine do not execute.
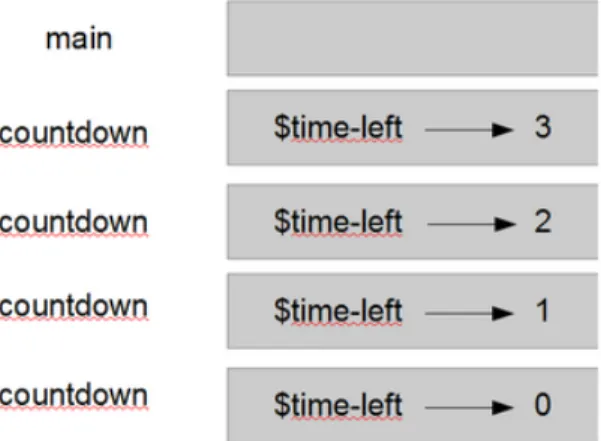
Fruitful Subroutines
- Return Values
- Incremental Development 71
- Incremental Development
- Composition 73
- Composition
- Boolean Functions
- A Complete Programming Language 75
- A Complete Programming Language
- More Recursion
- Leap of Faith 77
- Leap of Faith
- One More Example
- Checking Types 79
- Checking Types
- Multi Subroutines
- Debugging
- Glossary 83
- Glossary
- Exercises
The first step is to find the radius of the circle, which is the distance between the two points. But it's actually "Officially OK in Perl and Raku culture" to use the subset of the language you know.
Iteration
- Assignment Versus Equality
- Reassignment
- Updating Variables
- The while Statement 87
- The while Statement
- Local Variables and Variable Scoping 89
- Local Variables and Variable Scoping
- Control Flow Statements (last, next, etc.)
- Square Roots
- Algorithms 95
- Algorithms
- Debugging
- Glossary
- Exercises
- Exercises 97 Write a function called estimate-pi that uses this formula to compute and return an estimate of
In this case, you can use a control flow statement like property to break out of the loop. Otherwise, the program repeats whatever the user enters and returns to the top of the loop.
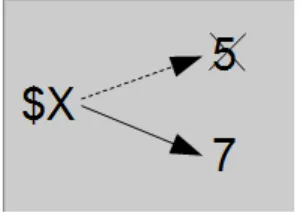
Strings
A String is a Sequence
But even if this can be useful sometimes, it's not the way you'd normally handle strings in Raku, which has higher-level tools that are more powerful and expressive, so you rarely need to use indexes or subscripts to access . individual characters. Also, if there is a real need to access and manipulate individual letters, it would make more sense to store them in an array, but we haven't covered arrays yet, so we'll have to come back to that later .
Common String Operators
- String Length
- Searching For a Substring Within the String
Again, the "nana" substring starts on the third letter of 'banana', but this letter is indexed 2, and the sixth letter is index 5. Common String Operators 101 Here the index is again an offset from the beginning of the string, so that the index of the.
Common String Operators 101 Here again, the index is an offset from the beginning of the string, so that the index of the
- Extracting a Substring from a String
- A Few Other Useful String Functions or Methods
- flip
- split
Here the star * can be thought of as representing the total size of the string;*-5 is therefore the position in the string five characters before the end of the string. So, substr(*-5) returns the characters from that position to the end of the string, i.e. the last five characters of the string.
Common String Operators 103
- String Concatenation
- Splitting on Words
- join
- Changing the Case
The join function takes a separator argument and a list of strings as arguments; it leaves them with the separator, concatenates everything into a single string, and returns the resulting string. In either case, wordsfirst splits the original string into a list of words and concatenates the elements of that list back into a new string concatenated with the separator.
String Traversal With a while or for Loop
String Traversal With a while or for Loop 105 This loop traverses the string and displays each letter on a line by itself. The loop condition
Looping and Counting
Regular Expressions (Regexes)
Using Regexes
Here, the regex engine starts by matching "bca" with bc, but that initial matching attempt fails because the next letter in the string, "b", does not match the "e" of the pattern. The regex engine backs off and starts searching again from the third letter ("c") of the string.
Building your Regex Patterns 109
Building your Regex Patterns
- Literal Matching
- Wildcards and Character Classes
- Quantifiers
You can also define your own character classes by inserting between<[ ]>any number of single characters and ranges of characters (expressed with two periods between the endpoints), with or without spaces. In this case, we use the “+” quantifier, which we'll discuss in the next section, but the point here is that you don't need to escape the dot and dash in the character class definition.
Building your Regex Patterns 111 The predefined quantifiers include
- Anchors and Assertions
- Anchors
- Look-Around Assertions
- Code Assertions
In case you are dealing with multi-line strings, you can also use ^^start of line and $$ end of line anchors. Look in the documentation (https: . //docs.raku.org/language/regexes#Look-around_assertions) if you need further details.
Building your Regex Patterns 113
- Alternation
- Grouping and Capturing
The difference between braces and square brackets is that braces don't group things together, they also contain data: they make the string that matches the braces available as a special variable (and also as an element of the resulting match object :)). Using square brackets when there is no need to wrap text has the advantage of not clogging up $0,$1,$2, etc.
Building your Regex Patterns 115
- Exercises on Regexes
The first word at the beginning of a string (for the purposes of these small exercises, the word separator can be thought of as a space, but you can do without this assumption);.
Putting It All Together
- Extracting Dates
Putting It All Together 117 Note that using the tilde as a prefix above leads $year, $month, and $day to be pop-
- Extracting an IP Address
With this definition of the $octet pattern, the regex will match any one- or two-digit number, or a three-digit number starting with the digits 1 through 2. But that's not good enough if we really want to check that the IP address is valid (for example, it would incorrectly accept 276 as a valid octet).
Substitutions
This definition of $octet again illustrates how the abundant use of whitespace and comments can help clarify intent.
Substitutions 119
- The subst Method
- The s/search/replace/ Construct
- Using Captures
- Adverbs
If the regex on the left contains catches, the replacement part on the right can use $O, $1, $2, etc. The most common modifiers used in substitutions are the :ignorecase (or :i) and :global(or :g) adverbs.
Debugging
A specific point to note here is that replacements are usually done only once.
Debugging 121
We've taken some license by arranging the variables in a box and adding dotted lines to show that the values $i and $ji represent the characters in $word1 and $word2. Starting with this diagram, run the program on paper and change the values of $i and $j between each iteration.
Glossary
Exercises 123Pattern A sequence of characters that uses a special syntax to describe the pattern from left to right A sequence of characters that uses a special syntax to describe from left to right.
Exercises 123 Pattern A sequence of characters using a special syntax to describe from left to right thePatternA sequence of characters using a special syntax to describe from left to right the
Exercises
Exercises 125 sub any_lowercase8(Str $string){
Case Study: Word Play
- Reading from and Writing to Files
- Reading Word Lists 129
- Reading Word Lists
- Exercises
- Search
- Words Longer Than 20 Characters (Solution)
- Search 131 Because the code is so simple, this is a typical example of a possible and useful one-liner
- Words with No “e” (Solution)
- Avoiding Other Letters (Solution)
- Search 133 here as an example; you might find it clearer to explicitly return values, except perhaps
- Using Only Some Letters (Solution)
- Using All Letters of a List (Solution)
- Alphabetic Order (Solution)
- Search 135 sub is_abecedarian (Str $word)
- Another Example of Reduction to a Previously Solved Problem
- Debugging
- Glossary
- Exercises
- Exercises 137 Write a program to find it
If one of the required letters is not in the word, we can return False. If we reach the end of the loop without finding an error, the word passes the test.
Arrays and Lists
Lists and Arrays Are Sequences
We'll devote most of the rest of this chapter to arrays rather than lists, but remember that many of the array functions and operators we'll study here also work on lists (at least most of the ones that wouldn't violate the immutability property of lists). The elements of an array (or list) do not have to be of the same type:.
Arrays Are Mutable
The end method returns the result of the element method minus one because, since indices start at 0, the index of the last element is one less than the number of elements. The unique function or method returns an array of unique elements of the input list or array (i.e. it returns the original list without any duplicate values):.
Adding New Elements to an Array or Removing Some 143
Adding New Elements to an Array or Removing Some
As you can see, when @add-array is added as an entity to the @numbersarray, @add-array becomes the new last item of the original array. There is also a prepend method that can replace unshift to append individual items of an array to the beginning of an existing array (instead of appending the array as a single entity).
Stacks and Queues 145
Stacks and Queues
Other Ways to Modify an Array
Other Ways to Modify an Array 147 my @digits = 1
Traversing a List
New Looping Constructs
The second new looping construct I want to introduce here uses the theloop keyword and is similar to C-styleforloop (that is, the C programming language loop). This special loop construct should probably only be used when the output state or the change made to the loop variable is unusual enough and would be difficult to express in a regular loop.
Map, Filter and Reduce 151
Map, Filter and Reduce
- Reducing a List to a Value
- The Reduction Metaoperator
- Mapping a List to Another List
- Filtering the Elements of a List
- Higher Order Functions and Functional Programming
More precisely, themapfunction iteratively assigns each element of the @lc_words array to the $_topical variable, applies the block of code after themapkeyword to $_ to create new values, and returns a list of these new values. Like map, the grep function iteratively assigns each element of the @input array to the $_topical variable, applies the code block after the grep keyword to $_ , and returns a list of the values that the code block evaluates to true.
Fixed-Size, Typed and Shaped Arrays 155 be thought of as generic abstract functions— they perform a purely technical operation:be thought of as generic abstract functions— they perform a purely technical operation
Fixed-Size, Typed and Shaped Arrays
Raku may not need to reallocate memory when you define the tenth array element. Specifying the type of elements and the maximum size of the array can lead to a noticeable increase in performance in terms of execution speed (at least for some operations) and significantly reduce the program's memory consumption, especially when working with large arrays.
Multidimensional Arrays
Sorting Arrays or Lists 157 my @temp;my @temp;
Sorting Arrays or Lists
Here it sorts all strings that start with an uppercase letter before any string that starts with a lowercase letter, which is probably not what you want.
More Advanced Sorting Techniques
More Advanced Sorting Techniques 159 The code block used here as the first sort argument uses the pa- placeholder.
More Advanced Sorting Techniques 159 The code block used here as the first argument to the sort routine uses the placeholder pa-
In all the examples above, the comparison subroutine accepted two parameters, the two items to be compared. Here, since the compare code block takes only one argument, it is intended to transform each of the items to be compared before running the standard cmprotien on the arguments.
Debugging 161
Debugging
Glossary
Exercises
For example, "depot" and "toped" form an inverted pair; other examples include "reward" and "load", or "desserts".
Hashes
- A Hash is a Mapping
- A Hash is a Mapping 167
- Common Operations on Hashes
- Hash as a Collection of Counters
- Looping and Hashes
- Reverse Lookup 171
- Reverse Lookup
- Testing for Existence
- Hash Keys Are Unique
- Hashes and Arrays
- Hashes and Arrays 175 sub invert-hash (%in-hash) {
- Memos
- Memos 177
- Hashes as Dispatch Tables
- Global Variables 179 it is possible to dynamically modify the behavior of a program after compile time, while itit is possible to dynamically modify the behavior of a program after compile time, while it
- Global Variables
- Debugging
- Glossary
The order of the key-value pairs is usually not the order in which you filled in the hash. The key is always mapped to the value, so the order of the elements doesn't matter.