Slides for Chapter 13: Transactions and Concurrency Control - Chapter 13 slides
Teks penuh
Gambar
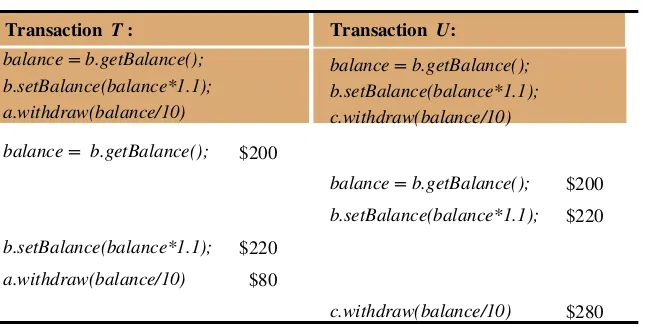
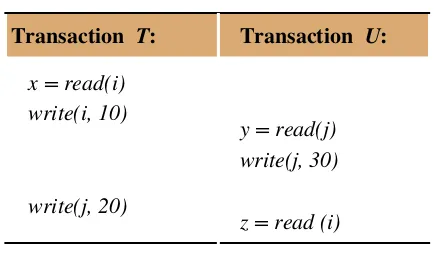

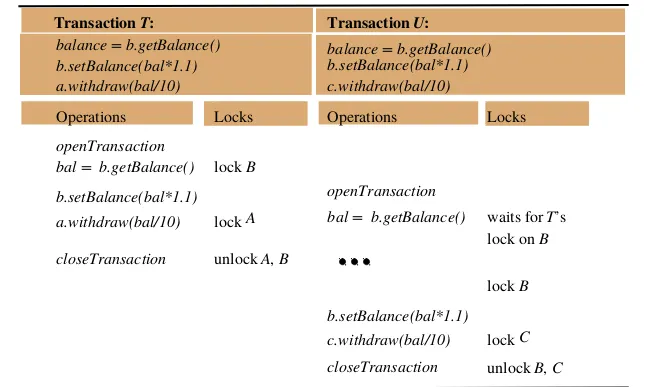
Dokumen terkait
dengan judul “Formulasi Emulgel Anti Acne Ekstrak Kulit Buah Manggis ( Garcinia mangostana L.): Pengaruh Kecepatan Putar pada Proses Pencampuran.. terhadap Sifat
[r]
Ketiga jenis mesin ini dibedakan berdasarkan jenis kemasan (merk) produk akhir yang diinginkan dan tahapan proses yang terjadi pada mesin-mesin
“Barangsiapa yang membacanya dalam sehari sebanyak 100 x, maka itu seperti membebaskan 10 orang budak, dicatat baginya 100 kebaikan, dihapus baginya 100 kesalahan, dirinya
As good children we ought to help our parents, you don’t have to do a hard thing just do simple things, probably wipe the floor, wash their clothes, or perhaps another
Abstrak : Permasalahan yang selalu dihadapi oleh guru pada saat pembelajaran adalah bola kasti siswa pada saat melakukan permainanya diganti dengan bola plastik
Puji syukur penulis panjatkan kehadirat Allah SWT, yang telah melimpahkan rahmat dan hidayah-Nya sehingga penyusunan tugas akhir dengan judul “Peranan Sistem Informasi
Puji Syukur penulis panjatkan kepada Tuhan Yang Maha Esa atas segala rahmat dan karunia-Nya, sehingga penulis dapat menyelesaikan Skripsi yang berjudul “Analisis dan